How to get record type id in salesforce
A typical use case for salesforce developers is to dynamically get record type id from salesforce to either use in apex, lightning web components (lwc) based on the record type name.
Here we have compiled a list of code snippets developers can use in various use-cases.
Get salesforce record type id
Find record type id from setup menu
The record type id can be retrieved from the setup menu. The record type page will have its id in the url.
For example for account, navigate to set up -> Object Manager -> Account -> Record Types -> 'Company'
The characters after /RecordTypes/ in the url is the record type id
Find record type id in apex without using SOQL
Use this approach to get the record type for an object in apex. This does not use SOQL and you will be within SOQL query limits when you use this approach.
public static final companyRecTypeId =
Schema.SObjectType.Account.getRecordTypeInfosByName().get('Company').getRecordTypeId();
Note: This does consume system resources so use this outside of loops for a better performance.
Find record type id using SOQL
Use this approach when you want to quickly query a record type id from developer console/ workbench/ vs code. As this requires a SOQL query, it is not recommended to use in apex.
public static final companyRecTypeId =
[Select id,name from RecordType where RecordType.Name='Company' AND SobjectType='Account'];
Here is how it can be used in the developer console query editor window
Find record type id in LWC using uiObjectInfoApi
The lightning web components can retrieve record type information via uiObjectInfoApi, no apex code is necessary. The uiObjectInfoApi returns the whole object metadata information including fields, child relationships, dependent fields etc..
Here is the code to retrieve the record type info using uiObjectInfoApi
import { LightningElement,wire } from "lwc";
import { getObjectInfo } from "lightning/uiObjectInfoApi";
import ACCOUNT_OBJECT from "@salesforce/schema/Account";
export default class RecordFormWithRecordType extends LightningElement {
@wire(getObjectInfo, { objectApiName: ACCOUNT_OBJECT }) wireAccountData(objectInfo,error){
if(objectInfo){
let recordTypeInfo = objectInfo?.data?.recordTypeInfos;
if(recordTypeInfo){
let companyRecType = Object.keys(recordTypeInfo).find(rtype=>(recordTypeInfo[rtype].name === 'Company'));
}
}
}
}
What is Decodeforce?
Decodeforce is a dedicated platform aimed at helping Salesforce developers improve their Apex coding skills by solving real-world programming challenges
Solve a number of practice scenarios in DML, SOQL, trigger and many more on Decodeforce.
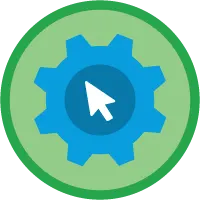
Collections & Control flow
Discover how to utilize various conditional statements and collection types in Apex..
Solve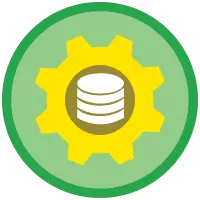
SOQL & DML
Explore SOQL queries, database manipulation statements (DML), and how to retrieve and update records in Apex.
Solve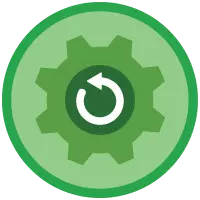
Apex Triggers
Master apex triggers by leveraging trigger events and context variables to address real business scenarios.
Solve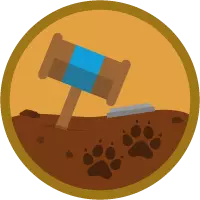
Governor limits
Resolve apex code that hits the governor limits and discover techniques to prevent such occurrences in your projects.
Solve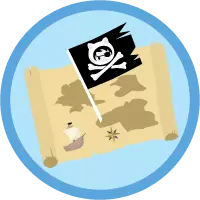
Apex REST API
Get hands-on experience with apex REST APIs to connect with external systems to send /receive data and updates records in Salesforce
Coming soon!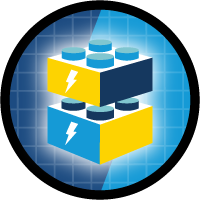
Lightning Web Components
Develop and get hands on experience in creating Lightning Web Components to create modern user interface for salesforce apps.
SolveTo know more click the Get started button below