How to get record id in lightning web components (lwc)
A typical use case for salesforce developers is to dynamically render lightning web components (lwc) based on the record id on lightning page, quick action or experience builder site pages.
Get record id
Get record id in lightning web components (lwc)
In order to retrieve the record id from the url, use @api recordId decorator.
Using @api recordId, the record id will be passed from the url to the lwc component.
//accountdetailsLWC.js
import { LightningElement, api } from "lwc";
export default class accountdetailsLWC extends LightningElement {
@api recordId;
}
//accountdetailsLWC.html
<template>
<lightning-record-form
record-id={recordId}
object-api-name="Account"
layout-type="Full"
mode="view">
</lightning-record-form>
</template>
When the component is rendered in the context of a record, the record id will be passed to the component by the lightning framework.
Note
The record id is automatically set only when used in an explicit record context.
Get record id in experience builder sites
The experience builder sites need additional configuration to get the current record id in the javascript file.
Once @api recordId is added in the javascript file, add a target config property in the meta.xml file. See an example given below.
//accountdetailsLWC.js-meta.xml
<targetConfigs>
<targetConfig targets="lightningCommunity__Default">
<property
name="recordId"
type="String"
label="Record Id"
default="{!recordId}"/>
</targetConfig>
</targetConfigs>
Get record id in a quick action lwc component
To get the record id for a quick action lwc component, add the following target, target configs in your component meta.xml file
//accountdetailsLWC.js-meta.xml
<targets>
<target>lightning__RecordAction</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordAction">
<actionType>Action</actionType>
</targetConfig>
</targetConfigs>
To see the official documentation for the different action type visit quick action section on lightning developers guide
How to get url parameters in experience builder pages
To retrieve the URL parameters in your LWC components, use the CurrentPageReference from lightning/navigation.
The platform stores the URL parameters in the state variable within the CurrentPageReference object.
To access a parameter named 'objectname', use the following code:
import { LightningElement, wire } from 'lwc';
import { CurrentPageReference } from 'lightning/navigation';
export default class accountdetailsLWC extends LightningElement {
@wire(CurrentPageReference) currentPageReference;
connectedCallback() {
let objectname = this.currentPageReference?.state?.objectname;
}
}
What is Decodeforce?
Decodeforce is a dedicated platform aimed at helping Salesforce developers improve their Apex coding skills by solving real-world programming challenges
Solve a number of practice scenarios in DML, SOQL, trigger and many more on Decodeforce.
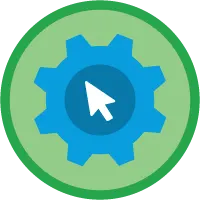
Collections & Control flow
Discover how to utilize various conditional statements and collection types in Apex..
Solve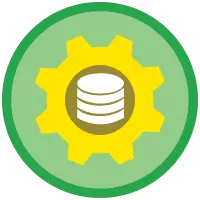
SOQL & DML
Explore SOQL queries, database manipulation statements (DML), and how to retrieve and update records in Apex.
Solve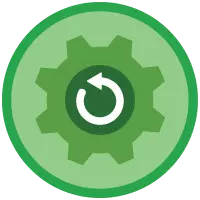
Apex Triggers
Master apex triggers by leveraging trigger events and context variables to address real business scenarios.
Solve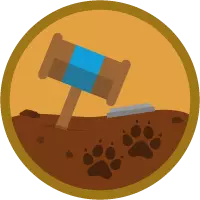
Governor limits
Resolve apex code that hits the governor limits and discover techniques to prevent such occurrences in your projects.
Solve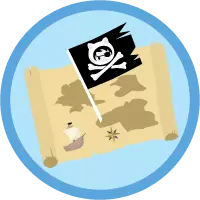
Apex REST API
Get hands-on experience with apex REST APIs to connect with external systems to send /receive data and updates records in Salesforce
Coming soon!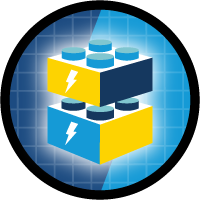
Lightning Web Components
Develop and get hands on experience in creating Lightning Web Components to create modern user interface for salesforce apps.
SolveTo know more click the Get started button below