Table of Contents
Batch apex in salesforce with examples
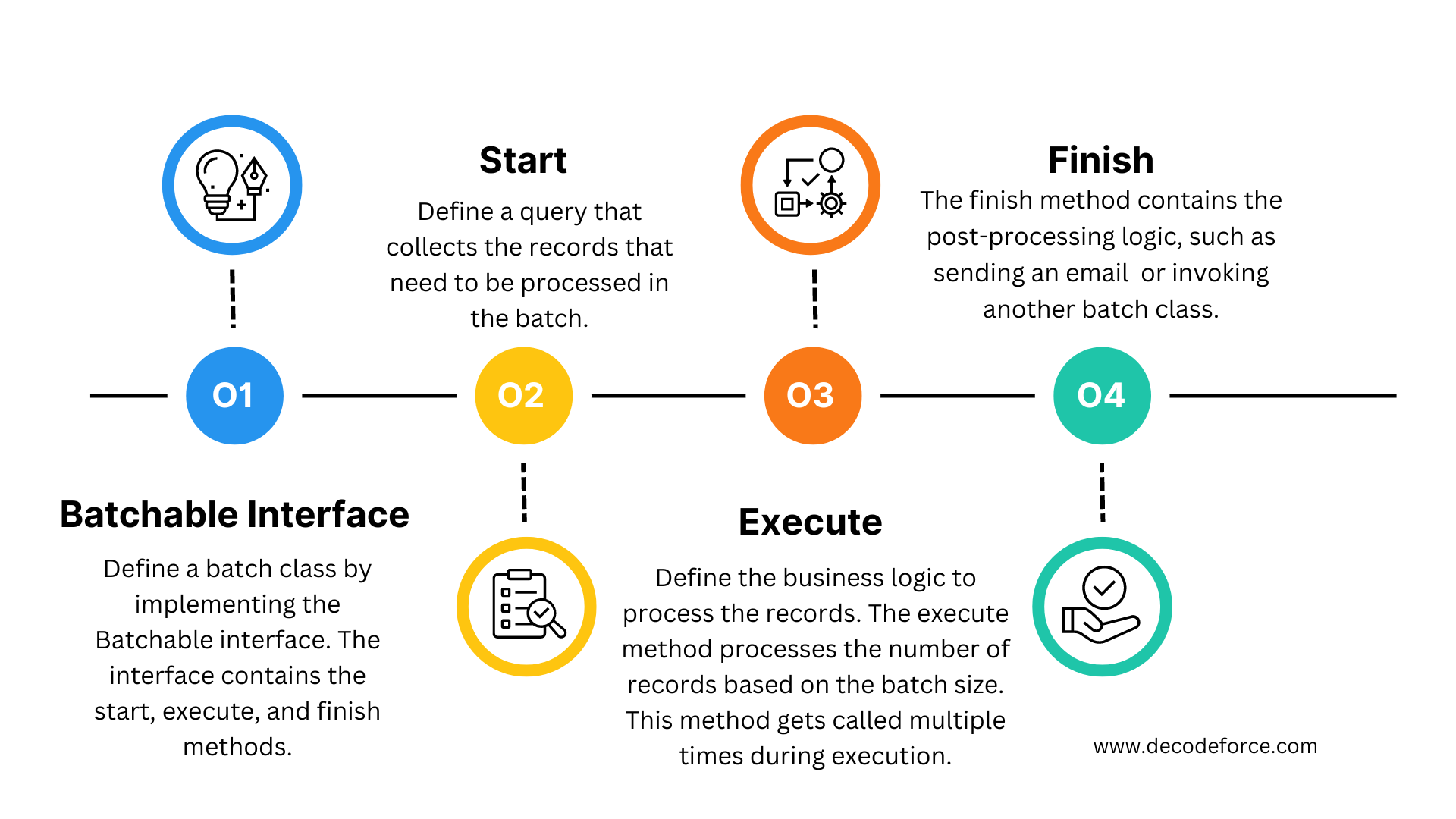
In the dynamic world of Salesforce, efficiently managing large datasets is crucial for maintaining optimal performance. Salesforce provides a powerful tool for this purpose: Batch Apex. This comprehensive guide delves into the details of Batch Apex, helping you understand its significance, implementation, and best practices.
What is a batch apex class
Batch Apex is a custom Apex class capable of processing large volumes of records in chunks, running with relaxed governor limits on the Salesforce platform. These classes can be scheduled to run daily or for repetitive jobs.
Why Use Batch Apex
Salesforce CRM is typically at the core of a business, and to ensure the platform runs optimally, some large volume record processing needs to be done asynchronously. With batch apex:
- Capability to Handle Large Data Volume:
The Apex batch class utilizes chunking of records to process a large volume of data efficiently.
- Performance optimization:
The Apex batch class operates in asynchronous mode, allowing the platform to process records when resources are available. This shift to asynchronous processing reduces the overall system load and enhances system performance.
- Ability to Schedule:
The Apex batch class can be scheduled to run at specific times, enabling processes to run at predetermined intervals such as daily, weekly, or monthly, depending on the schedule.
Key Elements of Batch Apex
Batch Apex utilizes the Database.Batchable interface provided by the Salesforce platform. This interface includes the following methods that need to be implemented:
- Start method
The start method returns a query locator responsible for identifying the records that need processing in the batch class. This method is called first and is invoked only once during the execution of a batch class.
- Execute method
The execute method receives the records that is returned from the start method query in chunks. The chunk size is the batch size defined when running/scheduling a batch class.
In real-world scenarios, the execute method may be executed multiple times depending on the volume of records returned by the start method and the batch size.
- Finish method
The finish method is utilized for performing post-processing steps, such as sending an email containing all processed or failed records once all execution steps are completed.
Additionally, the finish method can trigger another batch class if it needs to run immediately after the current batch class.
Implementing Batch Apex - An Example
global class AccountUpdateBatch implements Database.Batchable<SObject> {
global Database.QueryLocator start(Database.BatchableContext BC) {
// Query to fetch account records
String query = 'SELECT Id, Name FROM Account WHERE CreatedDate = TODAY';
return Database.getQueryLocator(query);
}
global void execute(Database.BatchableContext BC, List<Account> scope) {
// Process each batch of records
List<Account> accountUpdateList = new List<Account>();
for (Account acc : scope) {
acc.Description = 'Updated by system batch process';
accountUpdateList.add(acc);
}
if(accountUpdateList.size()>0){
update accountUpdateList;
}
}
global void finish(Database.BatchableContext BC) {
// Post-processing logic
System.debug('Batch Apex job completed successfully.');
}
}
Executing batch apex class from developer console
An Apex batch job can be executed from the Developer Console. To execute a batch class named 'AccountUpdateBatch', use the following code in the anonymous window of the Developer Console:
AccountUpdateBatch batchObj = new AccountUpdateBatch();
Database.executeBatch(batchObj, 200); //200 is the batch size
The above batch Apex program processes 200 records in the execute method and runs until all records in the start query are processed.
Scheduling batch apex class in salesforce
In order to schedule an apex class to run at specific intervals, first write an apex class that imlements the schedulable interface. The schedulable interface contains an execute method with a SchedulableContext parameter.
global class AccountUpdateBatch implements Database.Batchable<SObject>,Schedulable {
global void execute(SchedulableContext sc) {
AccountUpdateBatch batchObj = new AccountUpdateBatch();
Database.executeBatch(batchObj);
}
}
Alternatively use the System.scheduleBatch method without having to implement the Schedulable interface.
Apex batch job can be scheduled in following ways:
1. Schedule batch apex from developer console
To schedule a batch apex from developer console, execute the following code
AccountUpdateBatch batchObj = new AccountUpdateBatch();
String cronExp = '20 30 8 10 2 ?';
String jobID = System.schedule('Account Update Job', cronExp, batchObj);
2. Schedule batch apex from set up menu
To schedule an apex class from set up menu, go to set up -> apex class -> and click on the schedule apex button on the page. The system will open the following schedule apex page. Enter the name, apex class, start & end date, frequency to schedule the apex class to run in the specific intervals.
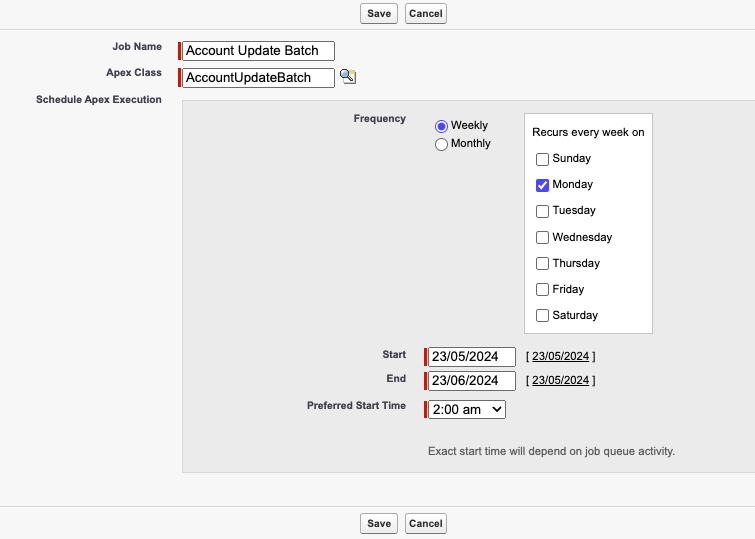
Monitoring Batch Jobs In Salesforce
To monitor the execution of a batch class in Apex, go to Setup ->Apex Jobs page. The Apex Jobs page will list the Batch Apex methods executed in the system with their respective statuses.

Chaining Batch Jobs
You can chain batch jobs by invoking another batch job from the finish method of the current one. This technique is beneficial for handling complex processes that need to be divided into multiple steps.
To run a contact update job after all accounts have been updated, call the contact update batch from the finish method of the account update batch. Refer to the sample code below.
global void finish(Database.BatchableContext BC) {
// Call second batch job
ContactUpdateBatch contactBatch = new ContactUpdateBatch();
Database.executeBatch(contactBatch);
}
Best practices
- Implement a handler class to process the records, rather than writing custom logic directly in the execute method.
- Take extreme care if you are scheduling a batch apex class from a trigger. You need to ensure that the trigger won't exceed the limit for scheduled classes. Pay special attention to API bulk updates, import wizards, mass record changes.
- To enable a batch class to make external callouts, implement the Database.AllowsCallouts interface.
- An org can only have 100 scheduled Apex jobs at one time.
- Salesforce schedules the class to run at the specified time, but the actual execution might be delayed due to service availability.
Conclusion
Batch Apex is an essential organizations for the efficient processing of large datasets within governor limits. Use Batch Apex effectively to keep your Salesforce applications robust, scalable, and efficient.
What is Decodeforce?
Decodeforce is a dedicated platform aimed at helping Salesforce developers improve their Apex coding skills by solving real-world programming challenges
Solve a number of practice scenarios in DML, SOQL, trigger and many more on Decodeforce.
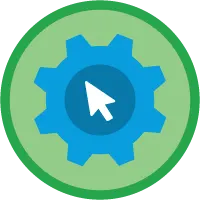
Collections & Control flow
Discover how to utilize various conditional statements and collection types in Apex..
Solve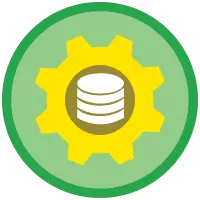
SOQL & DML
Explore SOQL queries, database manipulation statements (DML), and how to retrieve and update records in Apex.
Solve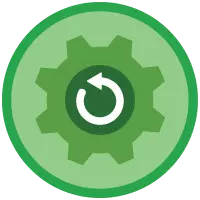
Apex Triggers
Master apex triggers by leveraging trigger events and context variables to address real business scenarios.
Solve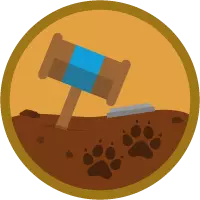
Governor limits
Resolve apex code that hits the governor limits and discover techniques to prevent such occurrences in your projects.
Solve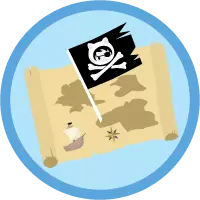
Apex REST API
Get hands-on experience with apex REST APIs to connect with external systems to send /receive data and updates records in Salesforce
Coming soon!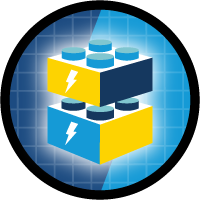
Lightning Web Components
Develop and get hands on experience in creating Lightning Web Components to create modern user interface for salesforce apps.
SolveTo know more click the Get started button below