Salesforce apex list class and methods with practice examples
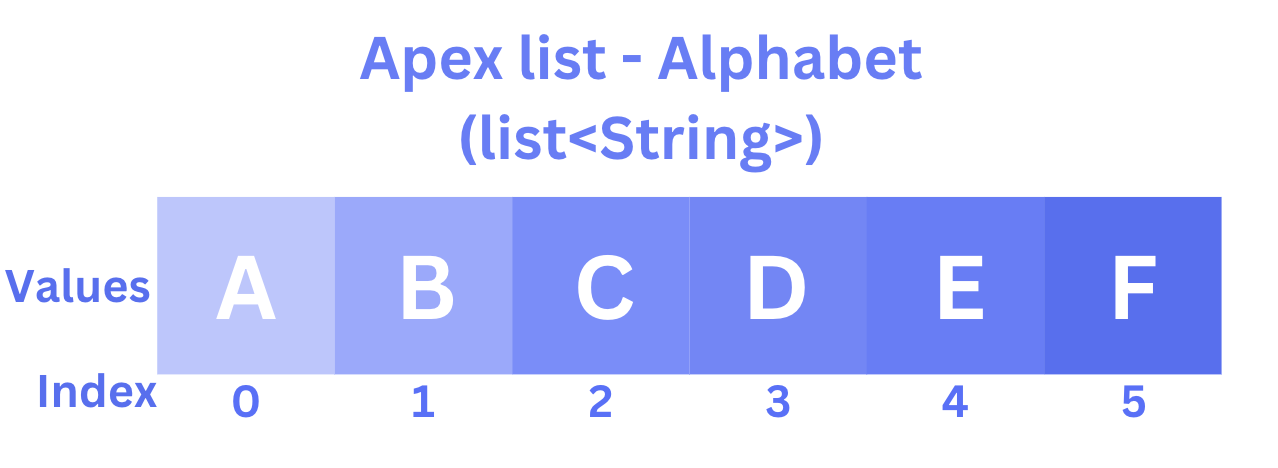
List is one of the collections in apex. A list is an ordered collection of elements, where each element can be accessed by its index. Apex lists class provides a number of methods to handle groups of records in salesforce.
In this guide, we will dive into list manipulation in salesforce apex, explaining essential list methods to use for real-world business scenarios.
Key topics
How to Create a List
To create a list, use the List keyword followed by angle brackets containing the data type.
Here is an example :
List<String> fruitList = new List<String>();
Note : Lists must always be initialized. If you operate on an uninitialized list, you may cause a null pointer exception.
What are the list methods
Salesforce apex list class provides a rich set of methods to manipulate lists. These methods allow developers to perform a variety of operations, such as adding, removing, sorting, and searching elements within a list.
The following are some of the most important list class methods available in Salesforce.
1. add
This method adds an element to the end of the list.
List<String> names = new List<String>{'Alice', 'Bob'};
names.add('Charlie');
System.debug('Names: ' + names); //Names: (Alice, Bob, Charlie)
2. addAll
This method adds all the elements from another list to the end of the current list.
List<String> names = new List<String>{'Alice', 'Bob'};
List<String> moreNames = new List<String>{'David', 'Eve'};
names.addAll(moreNames);
System.debug('Names after addAll: ' + names); //Names after addAll: (Alice, Bob, David, Eve)
3. clear
This method removes all elements from the list.
List<Integer> numbers = new List<Integer>{1, 2, 3, 4, 5};
numbers.clear();
System.debug('List after clear(): ' + numbers); // List after clear(): ()
4. contains
This method returns true if the list contains the specified element, otherwise it returns false.
List<String> fruits = new List<String>{'Apple', 'Banana'};
Boolean containsBanana = fruits.contains('Banana');
System.debug('Contains Banana: ' + containsBanana ); //Contains Banana: true
5. get
This method returns the element at the specified index in the list.
List<String> fruits = new List<String>{'Apple', 'Banana'};
String secondFruit = fruits.get(1);
System.debug('The fruit at index 1 is: ' + secondFruit); //The fruit at index 1 is: Banana
6. indexof
This method returns the index of the first occurrence of the specified element in the list, or-1 if the element is not found.
List<String> fruits = new List<String>{'Apple', 'Banana', 'Cherry'};
Integer missingIndex = fruits.indexOf('Grape');
System.debug('The index of Grape is: ' + missingIndex); //The index of Grape is: -1
7. isEmpty
This method returns true if the list is empty,otherwise it returns false.
List<Integer> numbers = new List<Integer>();
Boolean isListEmpty = numbers.isEmpty();
System.debug('Is the list empty? ' + isListEmpty); // Is the list empty? true
8. remove
This method removes the first occurrence of the specified element from the list.
List<Integer> numbers = new List<Integer>{10, 20, 30, 40, 50};
Integer removedElement = numbers.remove(2);
System.debug('List after removing by index: ' + numbers); //List after removing by index: (10, 20, 40, 50)
9. set
This method replaces the element at the specified index in the list with the specified element.
List<String> fruits = new List<String>{'Apple', 'Banana', 'Cherry', 'Date'};
fruits.set(1, 'Blueberry');
System.debug('List after use set(): ' + fruits); // List after use set(): (Apple, Blueberry, Cherry, Date)
10. size
This method returns the number of elements in the list.
List<String> fruits = new List<String>{'Apple', 'Banana', 'Cherry', 'Date'};
Integer listSize = fruits.size();
System.debug('The size of the list is: ' + listSize); //The size of the list is: 4
11. sort
This method sorts the elements in the list in ascending order, based on their natural ordering.
List<Integer> numbers = new List<Integer>{5, 3, 9, 1};
numbers.sort();
System.debug('Sorted numbers: ' + numbers); //Sorted numbers: (1, 3, 5, 9)
Exception - List has no rows for assignment to SObject
This exception happens when you try to use a value from a list that was created using a SOQL query, but the list is empty.
For example:
Account accRecord = [Select name from Account where Type = 'Prospect' ];
String accountName = accRecord.name;
The select query always returns a list. Always check if the list is empty before accessing the values from the list.
The modified code for the above scenario is :
List<Account> accRecords = [Select name from Account where Type = 'Prospect' ];
if(accRecords.size()>0){
String accountName = accRecord[0].name;
}
Exception - List index out of bounds
Apex throws a "List index out of bounds" exception when you try to access an element in a list using an index that doesn't exist in the list. Here is an example:
List<Integer> numbers = new List<Integer>{1, 2, 3};
//Throws List index out of bounds
Integer invalidNumber = numbers[4]; // Tries to access the 5th element (index 4), but the list only has 3 elements
To avoid this happening in your code, always check the list size before accessing an index.
Apex list practice examples
Following are a list of practice examples to learn and use the apex list methods. Click the following links to see the apex practice problems.
1. Add two string values to a list
Apex practice method to define and add two string values into a list.
2. Find the number of elements in a list
Apex practice method to find the number of elements in a list using apex list methods.
3. Insert an element at a specific index
Apex list practice example to add an element at specific index by using apex list methods.
4. Verify if an opportunity stage is in a list of final stages
Apex list method to verify if the opportunity stage belongs to one of the final stages.
5. Dedupe lead records in salesforce using apex
Learn to use apex list methods to deduplicate lead records in salesforce.
6. Find account ids from list of accounts
Retrieve account ids from any list of accounts using apex list methods.